Rsa Key Generation In Java
Java Program on RSA Algorithm
The simplest way to generate a key pair is to run ssh-keygen without arguments. In this case, it will prompt for the file in which to store keys. Here's an example: klar (11:39) ssh-keygen Generating public/private rsa key pair. RSA encryption in Java. We introduced the notion of asymmetric encryption, in which a key needed to encrypt data is made public, but the corresponding key needed to decrypt it is kept private, for example in a file on the server to which clients connect. Key generators are constructed using one of the getInstance class methods of this class. License key generator software free download. KeyGenerator objects are reusable, i.e., after a key has been generated, the same KeyGenerator object can be re-used to generate further keys. There are two ways to generate a key: in an algorithm-independent manner, and in an algorithm-specific manner.
RSA algorithm is an asymmetric cryptography algorithm. Asymmetric means that it works on two different keys i.e. Public Key and Private Key. As the name suggests that the Public Key is given to everyone and Private Key is kept private.
Key Generation. In lecture, we learned about RSA and how to implement RSA key generation in Java using the fields and methods of the BigInteger class. Problem 2: Write a Java method keyGenerate selects an n and calculates the values of k and d. Make two copies of your prelab code. Submit one copy to your Lab TA at the beginning of lab session. I want to generate 512 bit RSA keypair and then encode my public key as a string. RSA key pair and encode private as string. Generate RSA key pair in JAVA (in.
Algorithm
Step 1 : Choose two prime numbers p and q.
Step 2 : Calculate n = p*q
Step 3 : Calculate ϕ(n) = (p – 1) * (q – 1)
Step 4 : Choose e such that gcd(e , ϕ(n) ) = 1
Step 5 : Calculate d such that e*d mod ϕ(n) = 1
Step 6 : Public Key {e,n} Private Key {d,n}
Step 7 : Cipher text C = Pe mod n where P = plaintext
Step 8 : For Decryption D = Dd mod n where D will give back the plaintext.
If you need a dry run of the program or any other query, then kindly leave a comment in the comment box or mail me, I would be more than happy to help you.
Program
Rsa Key Generation In Java 10
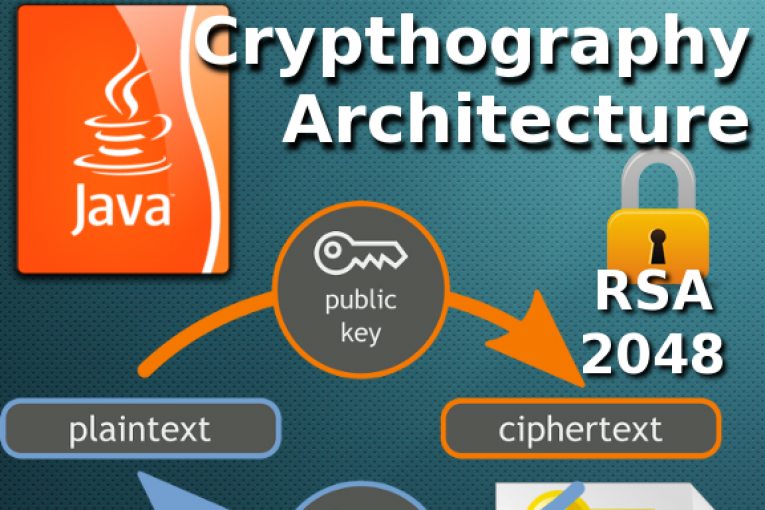
Rsa Key Generation In Java 7
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 | importjava.math.*; classRSA publicstaticvoidmain(Stringargs[]) Scanner sc=newScanner(System.in); System.out.println('Enter the number to be encrypted and decrypted'); doublec; System.out.println('Enter 1st prime number p'); System.out.println('Enter 2nd prime number q'); z=(p-1)*(q-1); { { } System.out.println('the value of e = '+e); { if(x%e0)//d is for private key exponent d=x/e; } System.out.println('the value of d = '+d); System.out.println('Encrypted message is : -'); //converting int value of n to BigInteger //converting float value of c to BigInteger msgback=(C.pow(d)).mod(N); System.out.println(msgback); } staticintgcd(inte,intz) if(e0) else } |